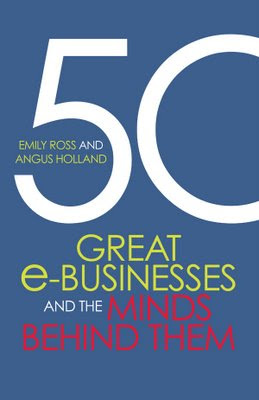
Great fun to read, highly recommended.
Sam Fu
Static members
Static constructor
using System;
namespace Console_CSharp
{
class TestStatic
{
static void Main(string[] args)
{
//----------Test_StaticField-----------
Console.WriteLine("{0},{1},{2}", Test_StaticField.x, Test_StaticField.y, Test_StaticField.z); //1,2,0
Test_StaticField t = new Test_StaticField(3);
Console.WriteLine("{0},{1},{2}", Test_StaticField.x, Test_StaticField.y, Test_StaticField.z); //3,3,3
//----------Test_StaticConstructor-----------
Console.WriteLine("{0},{1}", Test_StaticConstructor.x, Test_StaticConstructor.y); //100,200
//----------Test_StaticMethod-----------
Test_StaticMethod.Method(); //10,20
//----------Test_StaticProperty-----------
Test_StaticProperty.X = 20; //SET
int val = Test_StaticProperty.X; //GET
//----------TestInheritance-----------
Console.WriteLine(SubTest_Inheritance.x); //25
//----------Test_InheritanceHide-----------
SubTest_InheritanceHide.Method(); // Derived static method
Console.WriteLine(SubTest_InheritanceHide.x); //50
Console.ReadLine();
}
}
}
//--------------------------------------------------------------------------------------------------
class Test_StaticField
{
public static int x = 1;
public static int y = 2;
public static int z; //default value = 0 before initialization
public Test_StaticField(int i)
{
x = i; //normal constructor can access static members
y = i;
z = i;
}
}
//--------------------------------------------------------------------------------------------------
class Test_StaticConstructor
{
public static int x;
public static int y;
public int z; //default value = 0 before initialization
static Test_StaticConstructor() //static constructor is to initialize the static members
{
x = 100;
y = 200;
//z = 300; //compile error - static constructors can't access non-static data members
}
}
//--------------------------------------------------------------------------------------------------
class Test_StaticMethod
{
private static int x = 10;
private static int y = 20;
private int z = 30;
public static void Method()
{
Console.WriteLine("{0},{1}", x, y); //cannot access z here, static method can access only static members
}
}
//--------------------------------------------------------------------------------------------------
class Test_StaticProperty
{
public static int X
{
get
{
Console.WriteLine("GET");
return 10;
}
set
{
Console.WriteLine("SET");
}
}
}
//--------------------------------------------------------------------------------------------------
class Test_Inheritance
{
public static int x = 25;
}
class SubTest_Inheritance : Test_Inheritance
{
}
//--------------------------------------------------------------------------------------------------
class Test_InheritanceHide
{
public static int x = 25;
public static void Method()
{
Console.WriteLine("Base static method");
}
}
class SubTest_InheritanceHide : Test_InheritanceHide
{
public new static int x = 50;
//A static member can't be marked as override, virtual or abstract.
//However it is possible to hide a base class static method in a derived class by using the keyword new.
public new static void Method()
{
Console.WriteLine("Derived static method");
}
}
This article will talk about how to program a singleton into a fully lazily-loaded, thread-safe, simple and highly performant version: Implementing the Singleton Pattern in C#
window.onload = Window_Load;
function Window_Load()
{
txtTitle().value = "Title";
}
var mTitle = null;
function txtTitle()
{
if(mTitle == null)
{
mTitle = document.getElementById("txtTitle");
}
return mTitle;
}
C# example:
private Customers mCustomer = null;
private Customers Customer
{
get
{
if(this.mCustomer == null)
{
this.mCustomer = (Customers)ObjectBroker.GetObject(typeof(Customers),primaryKey);
}
return this.mCustomer;
}
}
private void Page_Load(Object sender, System.EventArgs e)
{
this.txtCustomer.Name = this.Customer.Name;
}
using System;
namespace DoFactory.GangOfFour.Singleton.Structural
{
class MainApp
{
static void Main()
{
// Constructor is protected -- cannot use new
Singleton s1 = Singleton.Instance(); //this will call: instance = new Singleton();
Singleton s2 = Singleton.Instance(); //this will use the reference created above
if (s1 == s2)
{
Console.WriteLine("Objects are the same instance");
}
Console.Read();
}
}
//-----------------------------------------------------------------------
class Singleton
{
//static field
private static Singleton instance; //use static because we don't want to create instance of the class
// protected constructor
protected Singleton()
{
}
//static method
public static Singleton Instance() //use static because we don't want to create instance of the class
{
// Uses lazy initialization
if (instance == null)
{
instance = new Singleton();
}
return instance;
}
}
}
This table may be used to populate the menu items on top of each web pages. Now if we want to order the menu items, we need to add a sequence column, so you may want to add 1,2,3... for each items in the menu table. Now what if we want to add an item in future that needs to be in between of these items, you will need to reorder a lot of the columns, so instead of add 1,2,3.. you can add 10,20,30.. so that you have more flexibility in the future. Another thing is that since the number of menu items will be very limited, the data type should be tinyint.
1) Int32 Structure
2) Byte Structure
[SerializableAttribute]
[ComVisibleAttribute(true)]
public struct Int32 : IComparable, IFormattable,
IConvertible, IComparable<int>, IEquatable<int>
[SerializableAttribute]
[ComVisibleAttribute(true)]
public struct Byte : IComparable, IFormattable,
IConvertible, IComparable<byte>, IEquatable<byte>
Various aspects of project management, including
What is a project management process?
Benefits of having processes in place:
Setting Up a Local Development Environment:
Setting Up a Remote Development Environment:
Here is a list of development tools/technology I have used or just know of:
Testing/Logging
Compare
Code generator
Screen Shot
.NET
Html Editor
Source Control
.NET Web Control
Print To Pdf
Regex
VB/C# converter
Build tools
The HttpSessionState object also supports the following methods:
Here are the things to note when using session:
Handling Session Events:
Controlling When a Session Times Out:
Using Cookieless Session State:
Cubes in Excel
Cubes in SSRS
Microsoft clients for SSAS
You can also develop custom clients
Where the data comes from
Criticality
Database Size
Concurrent Users
Response Time
Data Changes
Ad hoc querying
Querying complexity
Professional SQL Server 2000 Data Warehousing with Analysis Services
Why use OLAP?
Two kinds of tables form a data warehouse:
Fact tables
Dimension Tables
OLAP Schemas
Professional SQL Server Analysis Services 2005 with MDX
Professional SQL Server 2000 Data Warehousing with Analysis Services